With JSON-Map, you can map/convert JSON content using a JSONata expression.
JSONata is a very powerful query language that allows you to define functions, variables and lookup tables.
This makes it really easy to transform data according to your wishes.
Switch Fall 2022 and higher.
Use one of our sample flows and drop a sample file into the flow.
This app requires one incoming connection - more incoming connections are allowed.
The app supports traffic light outgoing connections of the following types:
Property | Value | Description |
---|---|---|
DataSource | Enum [Job | Dataset] | Defines the source of data. Only a valid JSON is supported. |
DatasetName | String | Name of the JSON dataset that should be mapped. |
WorkingMode | Enum [Multi-line | External File] | Defines if the jsonata expression is defined as multi-line field or as external file |
Bindings | String | Define a JSON object where each property can be accessed by $<key> in the JSONata expression. A binding is any value that's only available during runtime. E.g. { "variables": { "key1": "value1", "key2": "value2" } } |
LookupTables | String | Defines <key>=<value> pairs, where the value is the path to json file. The json file will be read and the content of the json is accessable by using $lookupTables.<key> in the jsonata expression. E.g.: formats=<pathToFormats JSON> |
LookupDatasets | String | Defines names of JSON datasets where the content is accessable by using $lookupTables.<datasetName> in the jsonata expression. Each dataset should be entered in a separate line. Make sure the dataset name is not equal to any other lookup table key. |
ResultDatasetName | String | Name of the resulting dataset. If a dataset with the same name already exists it will be overwritten. |
Property | Value | Description |
---|---|---|
MappingJsonataMultiline | String | Define a valid JSONata expression that should be used for mapping. |
Property | Value | Description |
---|---|---|
MappingJsonataFile | String | Path to a file that contains a valid JSONata expression. |
The documentation for JSONata is well written and there is also an exerciser which is highly recommanded to be used for testing expressions before using them in Switch. The exerciser gives you instant feedback!
Please note that the extended functions are only available in the Enfocus Switch environment and not in the exerciser!
Documentation:
https://docs.jsonata.org/overview.html
Exerciser / Tester:
https://try.jsonata.org/
As powerful as JSONata is, it cannot cover all functions. Therefore we have added some functions to JSONata which may be helpful in an Enfocus Switch environment.
NPM Path module:
All functions of the NPM module are available as JSONata function.
For example use $path.delimiter to get the delimiter of the operating system.
Check out the following link for more details https://nodejs.org/docs/latest/api/path.html
NPM UUID module:
The following functions of the NPM module are available as JSONata function:
For example use $uuid.v1() to call the v1 function of the module.
Check out the following link for more details https://www.npmjs.com/package/uuid
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
JSONata expression
This expression joins the value of the properties FirstName
and Surname
and searches for the phone number of type
mobile.
{
"name": FirstName & " " & Surname,
"mobile": Phone[type = "mobile"].number
}
Result
{
"name": "Fred Smith",
"mobile": "077 7700 1234"
}
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
JSONata expression
The following expression defines a variable fullName
which is then used in the resulting object.
(
$fullName := FirstName & " " & Surname;
{
"name": $fullName,
"mobile": Phone[type = "mobile"].number
}
)
Result
{
"name": "Fred Smith",
"mobile": "077 7700 1234"
}
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
JSONata expression
The following expression uses a separate function to get a phone number from the type.
(
$getPhoneNumberFromType := function($type) {(
$$.Phone[type = $type].number
)};
{
"name": FirstName & " " & Surname,
"mobile": $getPhoneNumberFromType('mobile')
}
)
Result
{
"name": "Fred Smith",
"mobile": "077 7700 1234"
}
If you need to pass a variable to the JSONata expression, bindings can be used. A binding can be accessed by using $ in the expression.
While this may not make much sense when using the multiline mode, it is very helpful if the expression is defined in an external file.
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
JSONata expression
This expression joins the value of the properties FirstName
and Surname
and searches for the phone number of type
mobile.
{
"name": FirstName & " " & Surname,
"mobile": Phone[type = "mobile"].number,
"jobName": $variables.jobName
}
Bindings
{
"variables": {
"jobName": "[Job.Name]"
}
}
Result
{
"name": "Fred Smith",
"mobile": "077 7700 1234",
"jobName": "contacts.json"
}
A lookup table can be accessed by using $lookupTables. in the expression.
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
Lookup JSON
How a lookup table is defined in the app:
Content of lookup table:
[
{
"customerRefId": 1,
"street": "2 Long Road",
"city": "Winchester",
"postcode": "SO22 5PU"
},
{
"customerRefId": 2,
"street": "56 Letsby Avenue",
"city": "Winchester",
"postcode": "SO22 4WD"
},
{
"customerRefId": 3,
"street": "1 Preddy Gate",
"city": "Southampton",
"postcode": "SO14 0MG"
}
]
JSONata expression
JSONata searches for an element in the lookup table where 'customerRefId' is equal to the 'ID' of the input JSON.
{
"name": FirstName & " " & Surname,
"mobile": Phone[type = "mobile"].number,
"address": $lookupTables.addresses[customerRefId=$$.ID]
}
Result
{
"name": "Fred Smith",
"mobile": "077 7700 1234",
"address": {
"customerRefId": 1,
"street": "2 Long Road",
"city": "Winchester",
"postcode": "SO22 5PU"
}
}
Input JSON
{
"ID": 1,
"FirstName": "Fred",
"Surname": "Smith",
"Age": 28,
"Phone": [
{
"type": "home",
"number": "0203 544 1234"
},
{
"type": "office",
"number": "01962 001234"
},
{
"type": "office",
"number": "01962 001235"
},
{
"type": "mobile",
"number": "077 7700 1234"
}
]
}
JSONata expression
This expression uses the v1
function of the uuid
module, which generates a UUID.
{
"id": $uuid.v1(),
"name": FirstName & " " & Surname,
"mobile": Phone[type = "mobile"].number
}
Result
{
"id": "8ce885c0-3df7-11ef-a6f6-c17f56ffa379",
"name": "Fred Smith",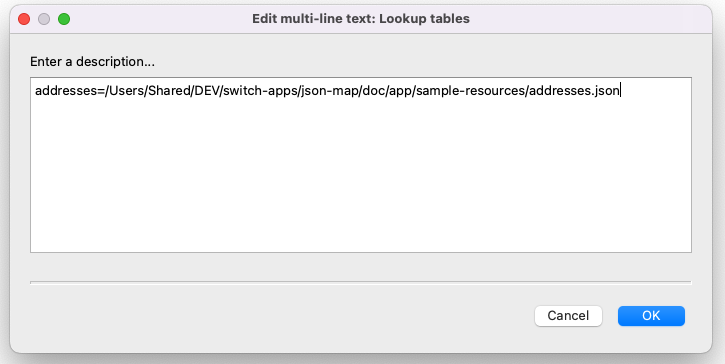
"mobile": "077 7700 1234"
}
This app uses two types of errors:
The following private data tags will be set if an error occurs:
Tag | Value | Type | Description |
---|---|---|
lastErrorElement | String | the name of the flow element |
lastErrorId | jsonCreateError | |
lastErrorCode | Number | an error code that defines the type of error that occured |
lastErrorMessage | String | detailed error message |
Error Codes:
enum ERROR_CODES {
generalError = 0,
fileHandlingError = 1,
fileFormatError = 2,
conversionError = 3,
invalidParameterValue = 4,
parsingError = 5,
}